jQuery to JavaScript Converter (Online tool + instructions)
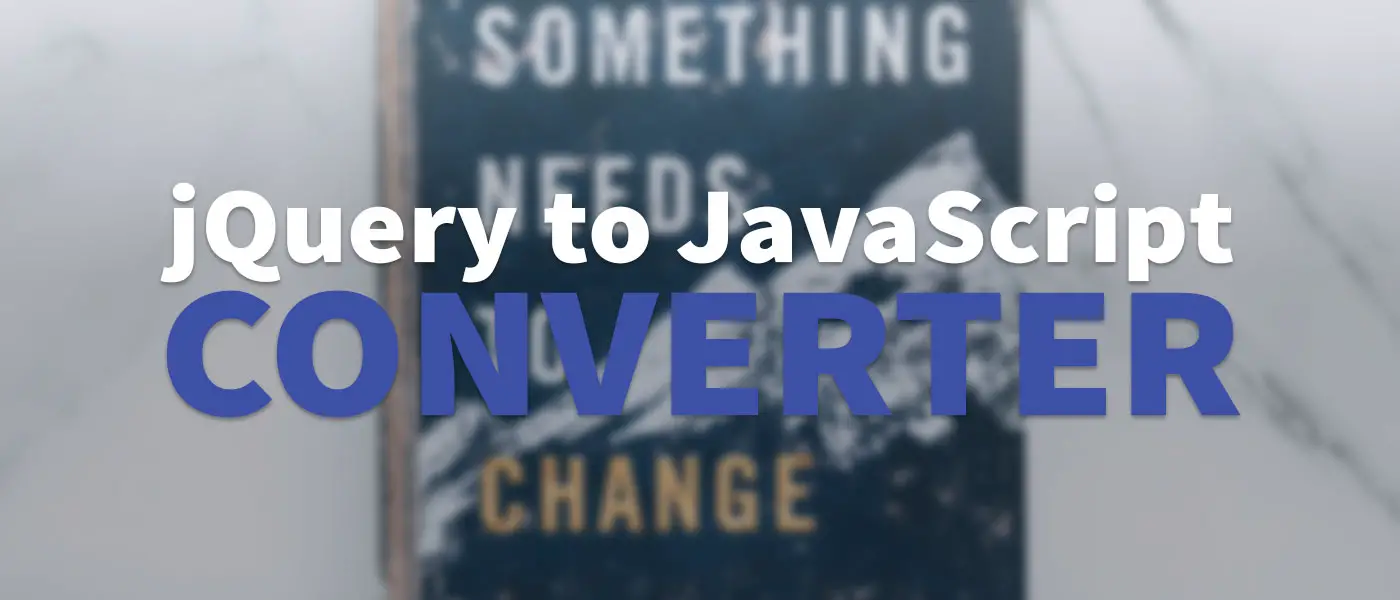
With my jQuery to JavaScript Converter you can quickly and easily convert from jQuery to vanilla JS.
You’ve already looked into switching from jQuery to pure JavaScript (also known as vanilla JS), otherwise you probably wouldn’t be here. That’s a good thing. I was also faced with this decision.
Online jQuery zu JavaScript Converter
The most important reason to come here: the jQuery to JavaScript converter 🎉
I have built you a tool/app to easily translate your jQuery code to JavaScript online. You can simply copy in your jQuery code, click on ‘Convert’ and get the pure JavaScript code directly. The conversion is performed directly in the browser, so your data remains local.
The app doesn’t work perfectly, which is why you should definitely read on, as I will give you important tips to achieve the best possible result.
But first, let’s take a look at why you should actually convert jQuery to JavaScript.
Improve SEO through Vanilla JavaScript?
Sounds funny at first, but there’s something to it. I’m always working on improving this site and the articles. For me – and also for search engines – the loading speed of the website is an important key figure.
I like to use PageSpeed Insights for testing. In the detailed report you get a detailed list of all problems, including the loading speed.
I had a too high value for the execution time of JavaScript. Now I am very satisfied with it.
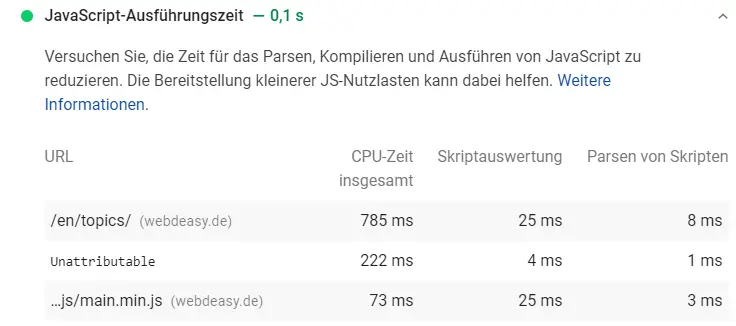
Also with resources that are loaded my jQuery file appeared additionally. And if you look here, the jQuery file with 11KB is already one of the larger files – despite being minified.
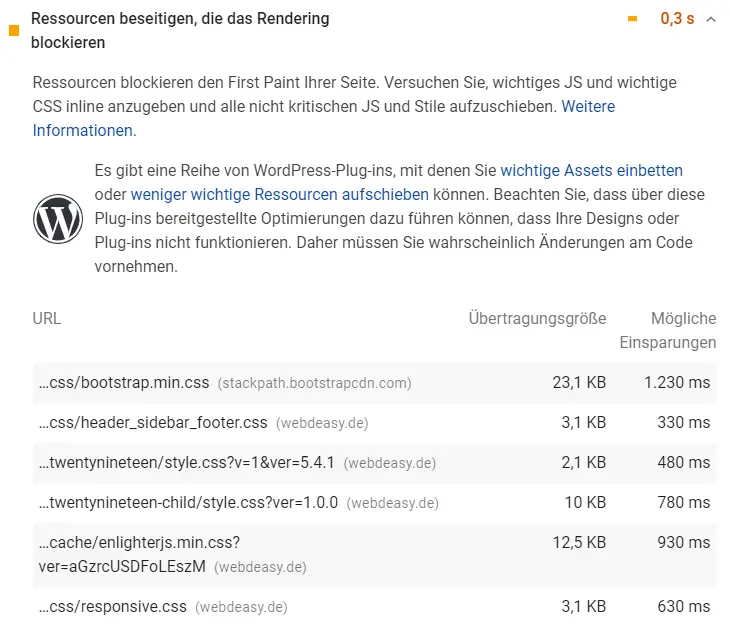
Don’t get me wrong, I love jQuery – simply an ingenious library. I have already programmed many great functions, like a Reading Position Indicator with jQuery.
But still I had to ban that from this site – for SEO and maybe also as a little challenge to deal more with Vanilla JS.
Now you know why we’re doing all this. So let’s start converting our code now. You should proceed in these steps:
- Remove dead code
- Use my online jQuery to JavaScript converter
- Get help from AI tools
- Convert remaining conversions using my 1:1 translation list
- Check and test code
- Done!
Remove dead code
If you already change something in the structure, you can use the opportunity directly to remove unnecessary and outdated code.
For this I made a list of all functions (there were 11 in total) and assigned a priority to each function.
I started with the important and essential functions and rewrote them in Vanilla JS.
Step by step. If you take over each function individually you might find errors or code that is no longer needed. You can delete them directly.
This will tidy up your new JavaScript file at the same time and it is also directly beneficial to the clarity.
An alternative to jQuery is Vue.js. Some functionalities can be implemented much easier and more dynamically with it. Vue.js also works without Build Tools – did you know that?
jQuery Code Conversion
Now you can use my jQuery to JavaScript converter to convert your code. Then come back to this point and transfer the result to an AI tool.
Since ChatGPT has found its way into the everyday life of developers, we also use this tool very regularly and we also take advantage of this for the conversion of jQuery code. However, since ChatGPT cannot process and create unlimited long texts, we have to keep a few things in mind. This approach has worked well for me:
- Locate logical code fragments: If you have a file with maybe 1000 lines of jQuery code, in the best case it is very modular, so here you can easily split the code into smaller pieces. These can be individual functions, for example.
- Convert jQuery code: Pass the individual code fragments to ChatGPT with the prompt “Rewrite this jQuery to Code pure Vanilla JS:
**CODE**
“.
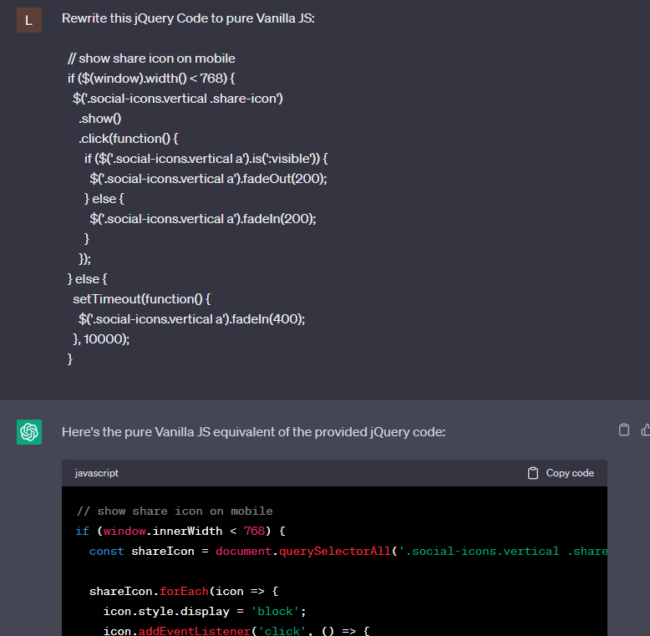
- Assemble JavaScript: Now take the generated code and put it step by step into a new JavaScript file.
- Check the code: Now you should look at the code again and refactor it a bit. Because ChatGPT is far from perfect! 🙂
1:1 jQuery to JavaScript translations
As I said, my converter app and ChatGPT don’t do the conversions perfectly. That’s why youmightnotneedjquery.com helped me as an additional resource. Direct functions in jQuery and JavaScript are compared there. I have expanded this list a little for you.
I hope that with the help of ChatGPT and my following compilation of 1:1 conversions you will be able to master your conversion from jQuery to JavaScript.
Document Ready Function
The function is called when the page is loaded. Thus, the execution of JavaScript does not block the page from loading.
// with jQuery $(function() { /* ... */ }); // Vanilla JS function ready(fn) { if (document.readyState != 'loading'){ fn(); } else { document.addEventListener('DOMContentLoaded', fn); } } ready(() => { /* ... */ });
Selecting elements
The biggest difference when selecting elements is that in pure JavaScript you have to create a loop if there are multiple elements. In jQuery, for example, you can register events using the selector and it applies to all of them. More details in the section Event Handling.
// with jQuery // multiple elements let buttons = $('button.red-btn'); // one element let button = $('button.green-btn'); // Vanilla JS // multiple elements let buttons = document.querySelectorAll('button.red-btn'); // one element let button = document.querySelector('button.green-btn');
With parentNode
you get the parent element. You can also string the statement together to get elements further up in the DOM – i.e. parentNode.parentNode
.
// with jQuery let foobarParent = $('#foobar-container').parent(); // Vanilla JS let foobarParent = document.querySelector('#foobar-container').parentNode;
Event Handling
For event handling you have to look if you have one or more elements. JQuery doesn’t care, it just registers the event. Here is an example for both situations.
// with jQuery $('.link').click(function() { /* ... */ }); // Vanilla JS // one element document.querySelector('#link').addEventListener('click', event => { /* ... */ }); // more elements document.querySelectorAll('.link').forEach(link => { link.addEventListener('click', event => { /* ... */ }); });
To deregister events the actual event callback function is necessary in Vanilla JS. So if you register an event, you should create it in a separate callback function.
// with jQuery $('#foobar').off('click'); // Vanilla JS document.getElementById('foobar').removeEventListener('click', clickEvent); function clickEvent(event) { }
Performing actions on elements
To achieve show()
and hide()
, we simply change the CSS property display
accordingly.
// with jQuery $('.foobar').show(); // Vanilla JS document.querySelectorAll('.foobar').forEach(element => { element.style.display = 'block'; });
// with jQuery $('.foobar').hide(); // Vanilla JS document.querySelectorAll('.foobar').forEach(element => { element.style.display = 'none'; });
Querying and changing attributes of elements
The query and handling of the classes works as easy as with jQuery. Only the spelling is a bit different. If you need a toggleClass()
function, you can easily program it yourself.
// with jQuery if($('.foo').hasClass('bar')) { /* ... */ } // Vanilla JS if(document.querySelector('.foo').classList.contains('bar')) { /* ... */ }
// with jQuery $('.foo').addClass('bar'); // Vanilla JS document.querySelector('.foo').classList.add('bar');
// with jQuery $('.foo').removeClass('bar'); // Vanilla JS document.querySelector('.foo').classList.remove('bar');
The query of other attributes is also quite similar. Note that sometimes the output is different from the jQuery function.
// with jQuery console.log($('.foobar').attr('checked')); // Output: checked/undefined // Vanilla JS console.log(document.querySelector('.foobar').checked); // Output: true/false
// with jQuery $('.foobar').html(); $('.foobar').html('<span>Hello world!</span>'); // Vanilla JS document.querySelector('.foobar').innerHTML; document.querySelector('.foobar').innerHTML = '<span>Hello world!</span>';
Determine offsets and dimensions
// with jQuery let offset = $(window).scrollTop(); // Vanilla JS let offset = window.pageYOffset;
// with jQuery $(window).width(); // Vanilla JS parseFloat(getComputedStyle(document.querySelector('html'), null).width.replace("px", ""));
// with jQuery $('.foobar').width(); // Vanilla JS document.querySelector('.foobar').offsetWidth;
// with jQuery $('.foobar').offset().top; // Vanilla JS document.querySelector('.foobar').offsetTop;
Styling of elements
For styles that are joined with a hyphen, you can use the Camel Case spelling. For example, justify-content
becomes justifyContent
.
// with jQuery $('.foobar').css('display', 'flex'); $('.foobar').css('margin-top', '3rem'); let displayProperty = $('.foobar').css('display'); // Vanilla JS document.querySelector('.foobar').style.display = 'flex'; document.querySelector('.foobar').style.marginTop = '3rem'; let element = document.querySelector('.foobar'); let elementStyle = getComputedStyle(element, null); let displayProperty = elementStyle.display;
Create new elements
If you want to create new elements and add them to an element, you can do this as follows.
Individual attributes and classes must be added individually, described in more detail in the section Querying and changing attributes of elements.
// with jQuery $('.foo').append('<p class="child" id="child-id">bar</p>'); // Vanilla JS let bar = document.createElement('p'); bar.classList.add('child'); bar.id = 'child-id'; bar.innerHTML = 'bar'; document.querySelector('.foo').appendChild(bar);
Manage Data Attributes
HTML data attributes help to assign more data to an HTML element than the element provides. Data attributes are appended as data-
attributes.
// with jQuery let action = $('#foo').data('action'); let customAttribute = $('#bar').data('custom-attribute-with-dashes'); // 'data-custom-attribute-with-dashes' in HTML // Vanilla JS let action = document.getElementById('foo').dataset.action let customAttribute = document.getElementById('bar').dataset.customAttributeWithDashes; // 'data-custom-attribute-with-dashes' in HTML
// with jQuery $('#foo').data('action', 'my-custom-action'); // Vanilla JS document.getElementById('foo').dataset.action = 'my-custom-action';
This is what the changeover looks like: Practical example
This is most of the features I needed when I switched over.
But I would like to give you exclusively the JavaScript file of this page. Before and after switching to Vanilla JS.
Maybe you can use the one or other line of code. 🙂
File before the change (jQuery)
$(function() { documentReady(); }); // WHEN DOCUMENT LOADED function documentReady() { // priavy container on visit begin if ( !getCookie('cookie-state') || getCookie('cookie-state') == undefined || getCookie('cookie-state') == '' ) { $('.privacy-popup').fadeIn(200); initCookieClicker(); } $('.open-cookie-menu').click(function() { $('.privacy-popup').fadeIn(200); initCookieClicker(); return false; }); // set perfect image ratio setImageRatio(); let resizeTimeout; $(window).resize(function() { clearTimeout(resizeTimeout); resizeTimeout = setTimeout(function() { setImageRatio(); }, 200); }); // remove ad containers setTimeout(function() { var adblockEnabled = false; // add test element and get its styles $('body').append('<div class="adBanner"></div>'); var adElement = document.getElementsByClassName('adBanner')[0]; var adElementStyle = getComputedStyle(adElement, null); if(adElementStyle.display === 'none') { // Adblock enabled $('.cwebdeasy-g-container').remove(); $('.webd-f-container').remove(); } }, 1500); $(window).scroll(function() { let offset = $(window).scrollTop(); if(offset > $('header#masthead').height()) { $('header#masthead').addClass('header-box-shadow'); } else { $('header#masthead').removeClass('header-box-shadow'); } }); // show share icon on mobile if ($(window).width() < 768) { $('.social-icons.vertical .share-icon') .show() .click(function() { if ($('.social-icons.vertical a').is(':visible')) { $('.social-icons.vertical a').fadeOut(200); } else { $('.social-icons.vertical a').fadeIn(200); } }); } else { setTimeout(function() { $('.social-icons.vertical a').fadeIn(400); }, 10000); } // collect menu data $('.menu-hauptmenue-container .sub-menu .menu-item a, .menu-hauptmenue-en-container .sub-menu .menu-item a').click(function() { _paq.push(['trackEvent', 'Desktop Menu', 'Click', 'Open: "' + $(this).html() + '"']); }); // collect sidebar click $('.menu-sidebar-tutorials-de-container li a, .menu-sidebar-tutorials-en-container li a').click(function() { _paq.push(['trackEvent', 'Sidebar Tutorials Menu', 'Click', 'Open: "' + $(this).html() + '"']); }); // collect sidebar click $('.sidebar-categories a, sidebar-categories a').click(function() { _paq.push(['trackEvent', 'Sidebar Categories Menu', 'Click', 'Open: "' + $(this).html() + '"']); }); // collect InPost Menu click $('.interesting-container li a').click(function() { _paq.push(['trackEvent', 'Interesting InPost Menu', 'Click', 'Open: "' + $(this).html() + '"']); }); // collect Table of contents click $('.table-of-contents li a').click(function() { _paq.push(['trackEvent', 'Table of contents', 'Click', 'Jump to: "' + $(this).html() + '"']); }); // open mobile menu let mobileMenuTimeout; $('.mobile-menu-button').click(function() { if (mobileMenuTimeout) return false; clearTimeout(mobileMenuTimeout); mobileMenuTimeout = setTimeout(function() { mobileMenuTimeout = null; }, 200); if ($(this).hasClass('open')) { $(this).removeClass('open'); $( '.menu-hauptmenue-container, .menu-hauptmenue-en-container' ).slideUp(200); $('.mobile-menu-bg-overlay').fadeOut(); } else { $(this).addClass('open'); $( '.menu-hauptmenue-container, .menu-hauptmenue-en-container' ).slideDown(200); $('.mobile-menu-bg-overlay').fadeIn(); $('.mobile-menu-bg-overlay') .off('click') .click(function() { $('.mobile-menu-button').click(); }); // open submenu if ( !$('.main-menu .submenu-expand') .siblings('.sub-menu') .is(':visible') ) { $('.main-menu .submenu-expand') // .first() .click(); } _paq.push(['trackEvent', 'Menu', 'Click', 'Open mobile menu']); } }); $('.main-menu .submenu-expand').click(function() { if ($(window).width() < 1200) { $(this) .siblings('.sub-menu') .slideToggle(); // _paq.push(['trackEvent', 'Menu', 'Click', 'Toggle submenu']); } }); // minimize code blocks $('.wp-block-code, .enlighter-default').each(function() { if ($(this).height() >= 450) { $(this) .children('code') .css('height', '450px'); $(this) .children('.enlighter') .css('display', 'block') .css('height', '470px'); $(this) .css('height', '545px'); $(this).append( '<span class="expand-code btn btn-block btn-sm btn-outline-primary mt-2">' + (lang === 'de' ? 'Aufklappen' : 'Expand') + '</span>' ); } }); // expand code $('.expand-code').click(function() { if ($(this).hasClass('open')) { $(this) .siblings('code') .css('height', '450px'); $(this) .siblings('.enlighter') .css('height', '470px'); $(this) .parent('.enlighter-default') .css('height', '545px'); $(this) .removeClass('open') .html(lang === 'de' ? 'Aufklappen' : 'Expand'); // _paq.push(['trackEvent', 'Code', 'Click', 'Minified View']); } else { $(this) .siblings('code') .css('height', 'auto'); $(this) .siblings('.enlighter') .css('height', 'auto'); $(this) .parent('.enlighter-default') .css('height', 'auto'); $(this) .addClass('open') .html(lang === 'de' ? 'Zuklappen' : 'Collapse'); // _paq.push(['trackEvent', 'Code', 'Click', 'Full View']); } return false; }); // smooth scroll on anchor click $('a[href^="#"]').click(function() { var elementName = $(this) .attr('href') .replace('#', ''); if(elementName == undefined || elementName == '') return false; var element = $('#' + elementName); if (!element.length) { return false; } var elementOffset = element.offset().top - 110; $('html, body').animate({ scrollTop: elementOffset }, 'slow'); location.replace('#' + elementName); return false; }); let sidebarScrollEnabled = true; let windowScrollTimeout; $(window).scroll(() => { clearTimeout(windowScrollTimeout); sidebarScrollEnabled = false; windowScrollTimeout = setTimeout(() => { sidebarScrollEnabled = true; }, 1000); }); setInterval(() => { if($(window).width() > 768 && sidebarScrollEnabled) { let offset = $(window).scrollTop(); if($('.single-after-content').offset().top < offset) return false; let adHeight = 0; if($('.cwebdeasy-g-container').length) adHeight = $('.cwebdeasy-g-container').height() + 120; $('.sidebar-outer-box .js-to-top-container').css('margin-top', (offset - adHeight - 50) + 'px'); } }, 5*1000); // lightbox $('figure > a > picture > img').click(function() { if ($(this).attr('src') != '') { $('.lightbox .img-container').html( '<div class="close">✖</div><img src="' + $(this).attr('src') + '"><p>' + $(this).attr('alt') + '</p>' ); $('.lightbox').fadeIn(); // _paq.push(['trackEvent', 'Lightbox', 'Click', 'Open Lightbox']); // register close event $('.lightbox .close, .lightbox .bg-overlay') .off('click') .click(function() { $('.lightbox').fadeOut(); }); } return false; }); } function initCookieClicker() { $('.privacy-popup .privacy-container #accept-cookies') .off('click') .click(function() { setCookie('cookie-state', 'all', 7); // matomo opt in _paq.push(['forgetUserOptOut']); // _paq.push(['trackEvent', 'Cookie Setting', 'Click', 'Accept all']); $('.privacy-popup').fadeOut(200); return false; }); $('.privacy-popup .privacy-container #necessary-cookies') .off('click') .click(function() { setCookie('cookie-state', 'necessary', 7); // _paq.push([ // 'trackEvent', // 'Cookie Setting', // 'Click', // 'Accept necessary' // ]); // matomo opt out _paq.push(['optUserOut']); // remove dark mode option // $('.dark-mode-switcher').remove(); // setCookie('theme', '', 0); $('.privacy-popup').fadeOut(200); return false; }); $('.privacy-popup .privacy-container #show-cookies') .off('click') .click(function() { $('.privacy-popup .more-cookie-infos').fadeToggle(); return false; }); } function setImageRatio() { // normal preview let imageElements = document.querySelectorAll('.post-preview-container .header-container'); if(imageElements.length) { let width = imageElements[0].offsetWidth; let height = width / 2; for(let i = 0; i < imageElements.length; i++) { imageElements[i].style.height = height + 'px'; } } // // small images let smallImageElements = document.querySelectorAll('.post-preview-container .horizontal-look > a'); if(smallImageElements.length) { width = smallImageElements[0].offsetWidth; height = width / 2; for(let i = 0; i < smallImageElements.length; i++) { smallImageElements[i].style.height = height + 'px'; } } } function getCookie(cname) { var name = cname + '='; var decodedCookie = decodeURIComponent(document.cookie); var ca = decodedCookie.split(';'); for (var i = 0; i < ca.length; i++) { var c = ca[i]; while (c.charAt(0) == ' ') { c = c.substring(1); } if (c.indexOf(name) == 0) { return c.substring(name.length, c.length); } } return ''; } function setCookie(cname, cvalue, exdays) { var d = new Date(); d.setTime(d.getTime() + exdays * 24 * 60 * 60 * 1000); var expires = 'expires=' + d.toUTCString(); document.cookie = cname + '=' + cvalue + ';' + expires + ';path=/'; }
File after the change (JavaScript)
let sidebarScrollEnabled = true; ready(() => { // priavy container on visit begin if (!getCookie('cookie-state') || getCookie('cookie-state') == undefined || getCookie('cookie-state') == '') { openCookieMenu(); } // open cookie menu document.querySelectorAll('.open-cookie-menu').forEach(cookieMenu => { cookieMenu.addEventListener('click', openCookieMenu); }); // set perfect image ratio setImageRatio(); // position share buttons positionSocialIcons(); // set perfect image ratio on resize let resizeTimeout; window.addEventListener('resize', () => { clearTimeout(resizeTimeout); resizeTimeout = setTimeout(() => { setImageRatio(); positionSocialIcons(); }, 200); }); // toggle mobile submenus document.querySelectorAll('.main-menu .submenu-expand').forEach(expander => { expander.addEventListener('click', toggleMobileSubMenus); }); // toggle mobile menu document.querySelector('.mobile-menu-button').addEventListener('click', toggleMobileMenu); // remove ad containers setTimeout(checkAdblock, 1500); // show shadow in header window.addEventListener('scroll', () => { toggleHeaderShadow(); }, {passive: true}); // sidebar scroll actions: TODO let windowScrollTimeout; window.addEventListener('scroll', () => { clearTimeout(windowScrollTimeout); sidebarScrollEnabled = false; windowScrollTimeout = setTimeout(() => { sidebarScrollEnabled = true; }, 1000); }, {passive: true}); setInterval(() => { execSidebarMovementActions(); }, 7*1000); // lightbox document.querySelectorAll('figure > a > picture > img, figure > a > img').forEach(lightboxLink => { lightboxLink.addEventListener('click', openLightbox); }); // show social icons showSocialIcons(parseFloat(getComputedStyle(document.querySelector('html'), null).width.replace("px", "")) <= 768); // collect matomo data registerMatomoEventListeners(); // minimize code blocks document.querySelectorAll('.wp-block-code, .enlighter-default').forEach(codeblock => { minimizeCodeblocks(codeblock); }); // scroll to (with offset) document.querySelectorAll('a[href^="#"').forEach(anchorLink => { anchorLink.addEventListener('click', anchorClick); }); // smooth scroll on page load setTimeout(() => { if(location.hash) { window.scrollTo(0, 0); let target = location.hash.split('#'); customScrollTo(document.getElementById(target[1])); } }, 200); }); /* FUNCTION AREA */ function ready(fn) { if (document.readyState != 'loading'){ fn(); } else { document.addEventListener('DOMContentLoaded', fn); } } function positionSocialIcons() { let iconWrapper = document.querySelector('.social-icons-fixed .social-icons'); if(!iconWrapper) return false; if(parseFloat(getComputedStyle(document.querySelector('html'), null).width.replace("px", "")) < 768) { iconWrapper.style.left = 'inherit'; iconWrapper.style.top = 'inherit'; iconWrapper.style.bottom = '1rem'; iconWrapper.style.right = '1rem'; } else { let blogpost = document.getElementsByClassName('post')[0]; iconWrapper.style.right = 'inherit'; iconWrapper.style.bottom = 'inherit'; iconWrapper.style.left = (blogpost.offsetLeft - 60) + 'px'; iconWrapper.style.top = '100px'; } } function anchorClick(event) { event.preventDefault(); let hrefSplit = event.target.href.split('#'); let elementID = hrefSplit[1]; if(elementID == undefined || elementID == '') return false; let element = document.getElementById(elementID); customScrollTo(element); } function customScrollTo(element) { location.replace('#' + element.id); if (element == null) return false; var elementOffset = element.getBoundingClientRect().top + window.pageYOffset - 110 window.scrollTo({ top: elementOffset, behavior: 'smooth' }); } function expandCode(event) { event.preventDefault(); if(event.target.classList.contains('open')) { // minimize code event.target.parentNode.querySelector('.enlighter').style.height = '470px'; event.target.parentNode.style.height = '534px'; event.target.classList.remove('open'); event.target.innerHTML = (lang === 'de' ? 'Aufklappen' : 'Expand'); } else { // maximize code event.target.parentNode.querySelector('.enlighter').style.height = 'auto'; event.target.parentNode.style.height = 'auto'; event.target.classList.add('open'); event.target.innerHTML = (lang === 'de' ? 'Zuklappen' : 'Collapse'); } } function minimizeCodeblocks(codeblock) { if(codeblock.offsetHeight >= 450) { codeblock.querySelector('.enlighter').style.height = '470px'; codeblock.querySelector('.enlighter').style.display = 'block'; codeblock.style.height = '534px'; let expandButton = document.createElement('span'); expandButton.classList.add('expand-code'); expandButton.classList.add('btn'); expandButton.classList.add('btn-block'); expandButton.classList.add('btn-sm'); expandButton.classList.add('btn-primary'); expandButton.classList.add('mt-2'); expandButton.innerHTML = (lang === 'de' ? 'Aufklappen' : 'Expand'); expandButton.onclick = expandCode; codeblock.appendChild(expandButton); } } function registerMatomoEventListeners() { // collect menu data document.querySelectorAll('.menu-hauptmenue-container .sub-menu .menu-item a, .menu-hauptmenue-en-container .sub-menu .menu-item a').forEach(elem => { elem.addEventListener('click', () => { _paq.push(['trackEvent', 'Desktop Menu', 'Click', 'Open: "' + elem.innerHTML + '"']); }); }); // collect sidebar click document.querySelectorAll('.menu-sidebar-tutorials-de-container li a, .menu-sidebar-tutorials-en-container li a').forEach(elem => { elem.addEventListener('click', () => { _paq.push(['trackEvent', 'Sidebar Tutorials Menu', 'Click', 'Open: "' + elem.innerHTML + '"']); }); }); // collect sidebar click document.querySelectorAll('.sidebar-categories a, sidebar-categories a').forEach(elem => { elem.addEventListener('click', () => { _paq.push(['trackEvent', 'Sidebar Categories Menu', 'Click', 'Open: "' + elem.innerHTML + '"']); }); }); // collect InPost Menu click document.querySelectorAll('.interesting-container li a').forEach(elem => { elem.addEventListener('click', () => { _paq.push(['trackEvent', 'Interesting InPost Menu', 'Click', 'Open: "' + elem.innerHTML + '"']); }); }); // collect Table of contents click document.querySelectorAll('.table-of-contents li a').forEach(elem => { elem.addEventListener('click', () => { _paq.push(['trackEvent', 'Table of contents', 'Click', 'Jump to: "' + elem.innerHTML + '"']); }); }); // collect logo click document.querySelectorAll('.custom-logo-link').forEach(elem => { elem.addEventListener('click', () => { _paq.push(['trackEvent', 'Link', 'Click', 'Logo Click']); }); }); } function showSocialIcons(mobile) { if (mobile) { document.querySelectorAll('.social-icons.vertical .share-icon').forEach(icon => { icon.classList.add('wd-show'); icon.addEventListener('click', () => { document.querySelectorAll('.social-icons.vertical a').forEach(iconElement => { if(iconElement.classList.contains('wd-show')) { iconElement.classList.remove('wd-show'); } else { iconElement.classList.add('wd-show'); } }); }); }); } else { setTimeout(function() { document.querySelectorAll('.social-icons.vertical a').forEach(icon => { icon.classList.add('wd-show'); }); }, 5000); } } function openLightbox(event) { event.preventDefault(); if(event.target.src != '') { let lightboxContent = '<div class="close">✖</div><img src="' + event.target.src + '"><p>' + event.target.alt + '</p>'; let lightboxContainer = document.querySelector('.lightbox .img-container'); lightboxContainer.innerHTML = lightboxContent; document.querySelector('.lightbox').classList.add('wd-show'); // _paq.push(['trackEvent', 'Lightbox', 'Click', 'Open Lightbox']); let closeElements = document.querySelectorAll('.lightbox .close, .lightbox .bg-overlay'); closeElements.forEach(closeElement => { closeElement.removeEventListener('click', closeLightboxEvent); closeElement.addEventListener('click', closeLightboxEvent); }) } } function closeLightboxEvent() { document.querySelector('.lightbox').classList.remove('wd-show'); } function execSidebarMovementActions() { let jsToTopContainer = document.querySelector('.sidebar-outer-box .js-to-top-container'); if(parseFloat(getComputedStyle(document.querySelector('html'), null).width.replace("px", "")) > 1200 && sidebarScrollEnabled) { let offset = window.pageYOffset; let singleAfterContent = document.querySelector('.single-after-content'); let singleAfterContentViewport = singleAfterContent.getBoundingClientRect(); let singleAfterContentOffset = singleAfterContentViewport.top + window.scrollY; let margin = 110; let sidebarAd = document.querySelector('.sidebar-box.cwebdeasy-g-container'); if(sidebarAd) { let sidebarAdHeight = sidebarAd.offsetHeight; let sidebarAdOffsetBottom = sidebarAd.getBoundingClientRect().top + sidebarAdHeight; if(sidebarAd) margin += sidebarAdHeight; if(window.pageYOffset < margin) { jsToTopContainer.style.marginTop = '1rem'; } else { jsToTopContainer.style.marginTop = (window.pageYOffset - margin) + 'px'; } } else { jsToTopContainer.style.marginTop = (window.pageYOffset - margin) + 'px'; } } else if(parseFloat(getComputedStyle(document.querySelector('html'), null).width.replace("px", "")) <= 1200) { jsToTopContainer.style.marginTop = '0px'; } } function toggleHeaderShadow() { let offset = window.pageYOffset; let header = document.querySelector('header#masthead'); if(offset > header.offsetHeight) { header.classList.add('header-box-shadow'); } else { header.classList.remove('header-box-shadow'); } } function checkAdblock() { let adblockEnabled = false; let adCheckElement = document.createElement('div'); adCheckElement.classList.add('adBanner'); document.querySelector('body').appendChild(adCheckElement); let adElement = document.getElementsByClassName('adBanner')[0]; let adElementStyle = getComputedStyle(adElement, null); if(adElementStyle.display === 'none') { // Adblock enabled document.querySelectorAll('.cwebdeasy-g-container').forEach(adElement => { adElement.remove(); }); document.querySelectorAll('.webd-f-container').forEach(adElement => { adElement.remove(); }); } } function openCookieMenu() { document.querySelector('.privacy-popup').classList.add('wd-show'); initCookieClicker(); } function initCookieClicker() { let acceptCookiesButton = document.querySelector('.privacy-popup .privacy-container #accept-cookies'); let necessaryCookiesButton = document.querySelector('.privacy-popup .privacy-container #necessary-cookies'); let showCookiesButton = document.querySelector('.privacy-popup .privacy-container #show-cookies'); acceptCookiesButton.removeEventListener('click', acceptCookiesButtonAction); acceptCookiesButton.addEventListener('click', acceptCookiesButtonAction); necessaryCookiesButton.removeEventListener('click', necessaryCookiesButtonAction); necessaryCookiesButton.addEventListener('click', necessaryCookiesButtonAction); showCookiesButton.removeEventListener('click', showCookiesButtonAction); showCookiesButton.addEventListener('click', showCookiesButtonAction); } function acceptCookiesButtonAction() { setCookie('cookie-state', 'all', 7); _paq.push(['forgetUserOptOut']); document.querySelector('.privacy-popup').classList.remove('wd-show'); } function necessaryCookiesButtonAction() { setCookie('cookie-state', 'necessary', 7); _paq.push(['optUserOut']) document.querySelector('.privacy-popup').classList.remove('wd-show'); } function showCookiesButtonAction() { let cookieInfos = document.querySelector('.privacy-popup .more-cookie-infos'); if(cookieInfos.classList.contains('wd-show')) { cookieInfos.classList.remove('wd-show'); } else { cookieInfos.classList.add('wd-show'); } } function setImageRatio() { // normal preview let imageElements = document.querySelectorAll('.post-preview-container .header-container'); if(imageElements.length) { let width = imageElements[0].offsetWidth; let height = width / 2; for(let i = 0; i < imageElements.length; i++) { imageElements[i].style.height = height + 'px'; } } // // small images let smallImageElements = document.querySelectorAll('.post-preview-container .horizontal-look > a'); if(smallImageElements.length) { width = smallImageElements[0].offsetWidth; height = width / 2; for(let i = 0; i < smallImageElements.length; i++) { smallImageElements[i].style.height = height + 'px'; } } } function getCookie(cname) { var name = cname + '='; var decodedCookie = decodeURIComponent(document.cookie); var ca = decodedCookie.split(';'); for (var i = 0; i < ca.length; i++) { var c = ca[i]; while (c.charAt(0) == ' ') { c = c.substring(1); } if (c.indexOf(name) == 0) { return c.substring(name.length, c.length); } } return ''; } function setCookie(cname, cvalue, exdays) { var d = new Date(); d.setTime(d.getTime() + exdays * 24 * 60 * 60 * 1000); var expires = 'expires=' + d.toUTCString(); document.cookie = cname + '=' + cvalue + ';' + expires + ';path=/'; } function toggleMobileSubMenus(event) { event.preventDefault(); if(parseFloat(getComputedStyle(document.querySelector('html'), null).width.replace("px", "")) < 1200) { let childs = event.target.parentNode.parentNode.children; for(let i = 0; i < childs.length; i++) { if(childs[i].classList.contains('sub-menu')) { if(childs[i].classList.contains('wd-show')) { childs[i].classList.remove('wd-show'); } else { childs[i].classList.add('wd-show'); } } } } } function toggleMobileMenu(event) { event.preventDefault(); let button = document.querySelector('.mobile-menu-button'); let mobileMenuOverlay = document.querySelector('.mobile-menu-bg-overlay'); if(button.classList.contains('open')) { // close menu button.classList.remove('open'); document.querySelectorAll('.menu-hauptmenue-container, .menu-hauptmenue-en-container').forEach(menu => { menu.classList.remove('wd-show'); }); mobileMenuOverlay.classList.remove('wd-show'); mobileMenuOverlay.removeEventListener('click', mobileMenuOverlayClick); } else { // open menu button.classList.add('open'); document.querySelectorAll('.menu-hauptmenue-container, .menu-hauptmenue-en-container').forEach(menu => { menu.classList.add('wd-show'); }); mobileMenuOverlay.classList.add('wd-show'); mobileMenuOverlay.addEventListener('click', mobileMenuOverlayClick); _paq.push(['trackEvent', 'Menu', 'Click', 'Open mobile menu']); } } function mobileMenuOverlayClick() { var event = document.createEvent('HTMLEvents'); event.initEvent('click', true, false); document.querySelector('.mobile-menu-button').dispatchEvent(event); }
I think it also makes it clear what I mean by cleaning up code.
Of course, the goal should always be to make code as maintainable as possible. But such files grow historically and of course it’s my own fault that such a chaos arises…
Convert jQuery to JavaScript: Is it worth it?
In my eyes: Yes! As well as cleaning up my code, I also removed unnecessary functions directly. Visitors to your site will also be pleased. I thought it wouldn’t work without jQuery. But if you really want to get everything out of your site, this is a good step.
And with my jQuery to JavaScript converter and my tips, the transition is also a little easier 🙂
What did you think of this post?


-
Pingback: JSON in PHP and JavaScript: Read, Get, Send, Convert, etc.
-
Pingback: How to build Vue.js apps without Node, Webpack, npm, build tools or CLI
-
Pingback: 8 Free SEO Tools you really need for your Website in 2021
-
Pingback: Programming Vue.js Fullpage Scroll
-
Pingback: Top 3 Practical Examples to learn Vue.js