Programming Reading Position Indicator (CSS & JavaScript)
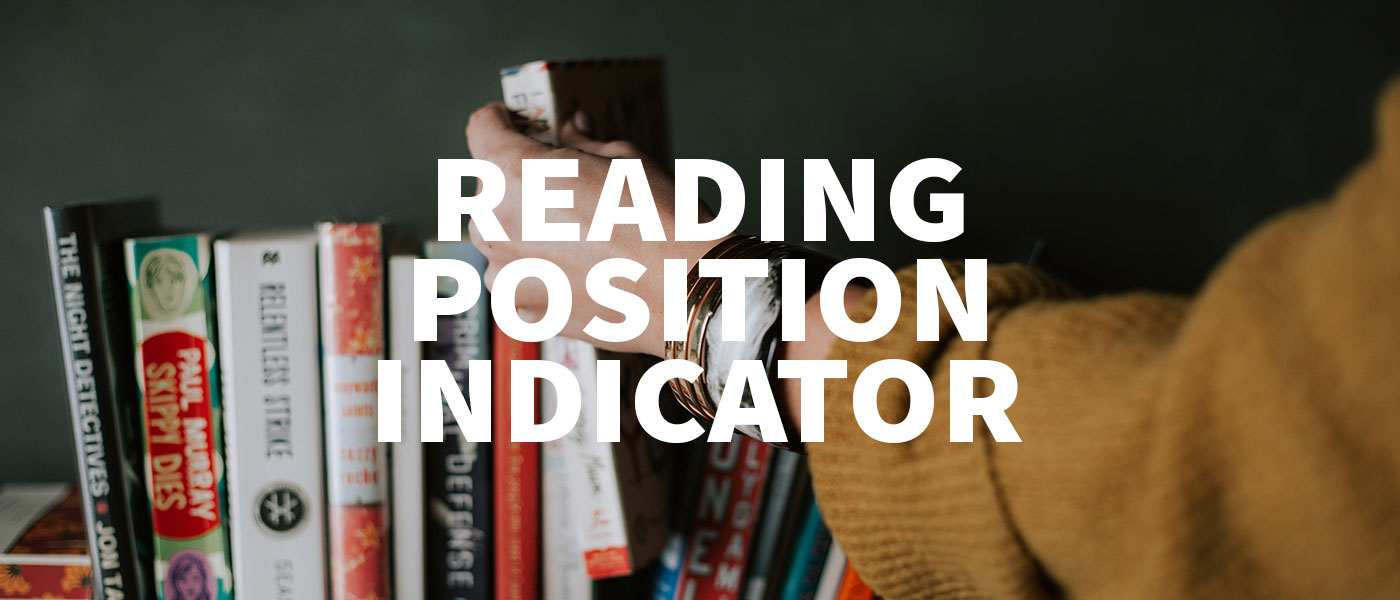
The Reading Position Indicator is a bar on your website that shows how much the visitor has already read of your content – and you can program it yourself so easily.
Reading Progress Bar, Scroll Position or Scroll Indicator: the mysterious bar has many names. A simple feature that gives your visitor an overview of how much they have already read of your post.
Especially for blogs a reading position indicator is often a nice thing. Of course, it also works completely responsive, so also for all mobile devices. You can try it out here.
The Reading Position Indicator is also easily integrated into WordPress. How to do that you will learn in the last step.
1. Design HTML structure
First we need the actual bar. For this we create a container, which we later set to full width and a container inside, which is always as long as the current progress should be.
<div id="read-progress" class="progress"> <div class="progress-bar"></div> </div>
2. Styling Reading Position Indicator (CSS)
If you have Bootstrap embedded in your site, you can use a ready-made progressbar and skip this step. You can find the link here.
Pssssst! Do you already know these CSS tricks?
The basic styling is done with these few lines. Here the bar is always at the top of your website.
.progress { width: 100%; position: fixed; top: 0; left: 0; height: 7px; background-color: #d3d3d3; } .progress > .progress-bar { height: 7px; width: 0px; background-color: #3d50a7; }
If you prefer to have the bar at the bottom you can use this CSS code.
.progress { width: 100%; position: fixed; top: calc(100vh - 7px); left: 0; height: 7px; background-color: #d3d3d3; } .progress > .progress-bar { height: 7px; width: 0px; background-color: #3d50a7; }
All parameters (thickness, color, …) you can adjust according to your wishes, of course.
3. Create functionality (JavaScript)
Now comes the most exciting and important part of the feature. For this we first include jQuery before the closing <body>
-tag and then the code for the bar.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
After that you can already paste this code into your JavaScript file or via tag after the jQuery inclusion.
$(function() { $(window).scroll(function() { let offset = $(window).scrollTop(); let windowHeight = $(window).height(); let height = $(document).height(); let progress = (offset / (height - windowHeight)) * 100; $("#read-progress .progress-bar").css("width", progress + "%"); }); });
For each scroll event, the code calculates the current progress in percent based on the height and scroll position and sets the colored bar to the corresponding width.
Many supposedly complex things can be programmed quite easily by yourself. For example, do you want to know how to program your own WYSIWYG editor?
Embed Reading Progress Bar in WordPress
If you are using WordPress you can of course also use this code. Just put the code in the following places:
HTML | footer.php or header.php |
CSS | style.css |
jQuery integration | footer.php (before closing <body> -tag) |
JavaScript | Any .js file or after jQuery integration |
If you have any questions about integration or functionality, feel free to post a comment.
What did you think of this post?


-
Pingback: From jQuery to JavaScript - How to make the move