Detect tab changes in the browser: JavaScript Blur & Focus
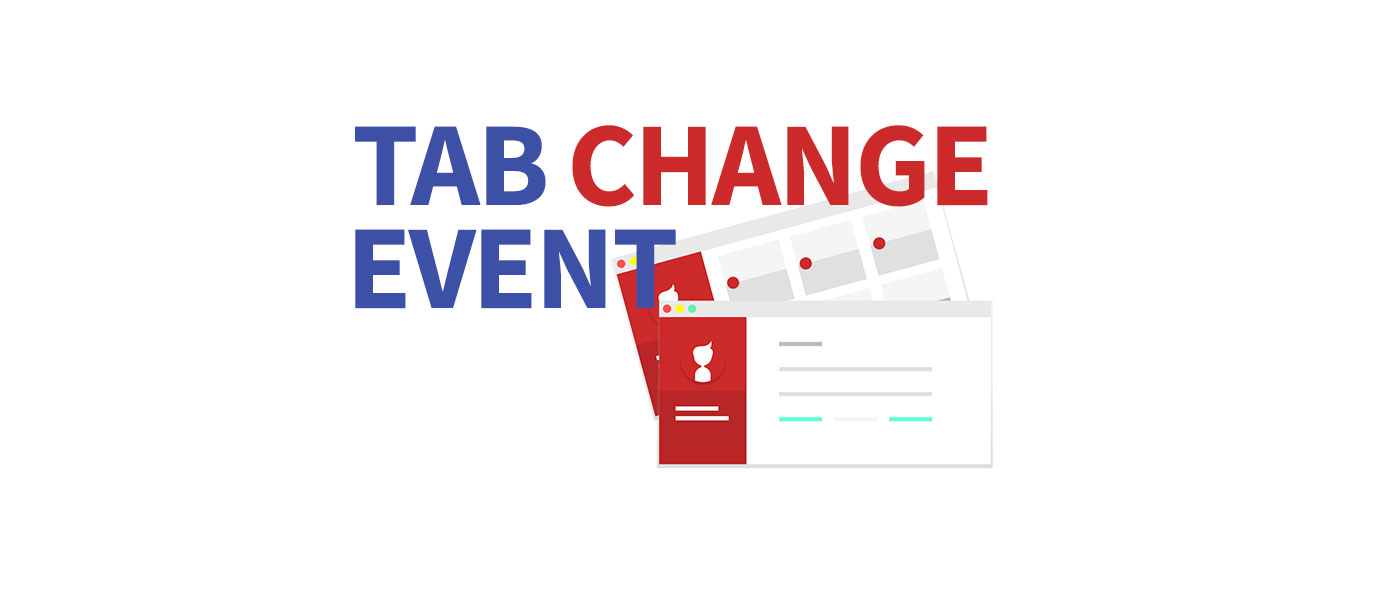
By detecting the tab change in the browser you can conjure up some cool functions. And you can find out how to do this here!
There are some features where it can be useful to be able to see the tab change of a visitor. These include limiting background activity and playing an animation on your website.
A nice feature that you can build by detecting the tab change is also this one:

The page title can be changed as desired and can display funny or helpful information. A little gimmick that can provide that special something and can also lead to some people being surprised.
Besides a fun effect, changing the page title can also attract the user’s attention to your page (again). If the user is inclined to leave the page, he can be “pulled” back to your page with a funny, nice or helpful hint.
I’ll also show you how to build the well-known WordPress preview feature yourself, so that the browser automatically switches tabs.
Reduce background activities
If you perform complex calculations with JavaScript, this will put a strain on your visitors’ browsers. But since we know when a visitor is on the tab of our website and when not, we can pause the calculations in the corresponding time period, as long as this does not affect the expected result.
By limiting these background activities, especially users with weaker computers or smartphones can be relieved.
There is (almost) always potential for optimization: With these Code Snippets for WordPress you can still tune your page properly!
Another field of application is the playing of an animation. If your visitor (luckily for you) returns to your site, you could greet him with a great animation. Of course, you should keep everything within limits so that it doesn’t get out of hand.
For serious sites this might be less appropriate… but if you want to prove your skills or it just fits to the theme of the site, why not!
Detect tab changes with JavaScript
To detect the tab change we use pure JavaScript without jQuery etc. Everything you need is hidden in this code.
If you want to switch from jQuery to pure JavaScript, this offers some advantages and you can still use all the great features. 🙂
We register the blur
event on the global document
variable. Generally, the blur
and focus
events are often used in conjunction.
The blur
event is triggered when an element or the entire tab (document) loses focus, i.e. when we no longer access it. In contrast, the focus
event is triggered when an element or the tab (document) is refocused, i.e. in our case it is back on the tab.
// user leaves the tab document.addEventListener('blur', (e) => { // your custom code here }); // user enters the tab (again) document.addEventListener('focus', (e) => { // your custom code here });
If you use jQuery you can use the same code, normal JavaScript works there too of course.
More about blur and focus can be found in the MDN web docs.
By the way: The demo shown at the beginning can be easily implemented with this code:
let siteTitle = ''; window.addEventListener('blur', () => { siteTitle = document.title; document.title = 'Come back! :c'; }); window.addEventListener('focus', () => { document.title = siteTitle; });
Automatic tab switching like in the WordPress preview
When you use the backend preview on WordPress it opens in a new tab. If you click again and the tab is still open, the browser switches back to this tab and reloads the URL. Here you can try it yourself:
The function can be implemented via two simple lines of code:
// the URL to open in the new tab let url = previewInput.value; // open tab or switch to tab if already exists let previewTab = (window.previewTab && window.previewTab.close()) || window.open(url, "previewTab");
I have chosen the name previewTab
freely. If you want to use another one, you can replace all occurrences of it in the code (4x).
This function has nothing to do with the blur & focus event, but I find this function so cool and simple and wanted to show it! 🙂
What did you think of this post?

