Programming interactive (rotatable) 3D cube
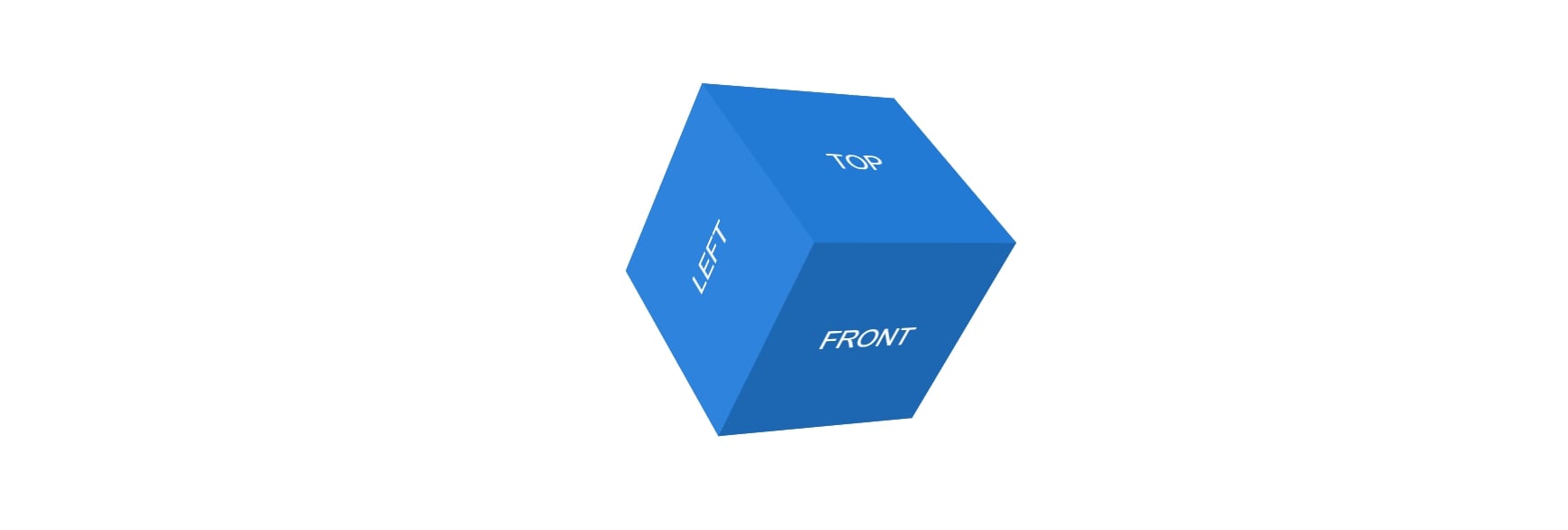
You move your cell phone and the cube turns? Yes! That’s exactly what we’re programming today. With the help of the devices alignment via JavaScript.
Demo
The following demo only works on mobile devices. On the desktop version, the 3D cube is simply displayed rigidly.
So grab your cell phone and look at the fancy cube!
The Cube
I created the cube with the help of these instructions: CSS 3D Cube. Nevertheless I would like to explain the implementation in my own words.
HTML
In HTML we have an outer container and an additional container in which the individual pages of the cube are located.
<div class="wrapper"> <div class="cube"> <div>Top</div> <div>Bottom</div> <div>Left</div> <div>Right</div> <div>Back</div> <div>Front</div> </div> </div>
CSS
Here we center the cube in the wrapper, specify the height and width and use the perspective
and transform-style
attributes to make the cube look like 3D.
.wrapper { height: 250px; perspective: 700px; -webkit-perspective: 700px; display: flex; justify-content: center; align-items: center; width: 100%; font-family: arial, sans-serif; } .cube { width: 100px; height: 100px; transform-style:preserve-3d; transform:rotateY(35deg) rotatex(50deg); }
Below we specify the height and width of the individual parts and center the text on the individual pages.
.cube > div { position: absolute; width: 120px; height: 120px; display: flex; justify-content: center; align-items: center; color: #FFF; text-transform: uppercase; }
Now we go through the individual pages and set the position and rotation. We have to set the Z-value to half the width: 120px/2 = 60px.
.cube div:nth-child(1) { transform:translateZ(60px); background:#237ad4; } .cube div:nth-child(2) { transform: rotateX(180deg) translateZ(60px); background:#2070c3; } .cube div:nth-child(3) { transform: rotateY(-90deg) translateZ(60px); background:#2e84dd; } .cube div:nth-child(4) { transform:rotateY(90deg) translateZ(60px); background:#3f8edf; } .cube div:nth-child(5) { transform: rotateX(90deg) translateZ(60px); background:#2070d3; } .cube div:nth-child(6) { transform:rotateX(-90deg) translateZ(60px); background:#1d67b2; }
Detect device orientation
The part that sounds hard at first is basically the simplest.
We can intercept the recognition of the orientation of the device via the JavaScript event deviceorientation
.
The value of the x-axis is the event variable gamma
and the value of the y-axis is the event variable beta
.
window.addEventListener("deviceorientation", (e) => { const beta = e.beta; const gamma = e.gamma; // disable for desktop devices if(beta == null || gamma == null) { return false; } document.getElementsByClassName('cube')[0].style.transform = 'rotateY(' + gamma*3 + 'deg) rotateX(' + beta*3 +'deg)'; }, true);
If the value of a variable is zero, it is a desktop device and we cancel the event.
If it is a mobile device, we set the variable for x and y and multiply it by 3 to adjust the rotation speed when moving the mobile device.
Docu to deviceorientation
: here.
Conclusion
The event deviceorientation
is very well suited for these, but also for many other purposes and unknown to many developers. Where would you like to use this function? Feedback and suggestions for improvement are welcome in the comments! 🙂
What did you think of this post?


Muchas gracias. ?Como puedo iniciar sesion?