8 CSS tricks you should know as a web developer
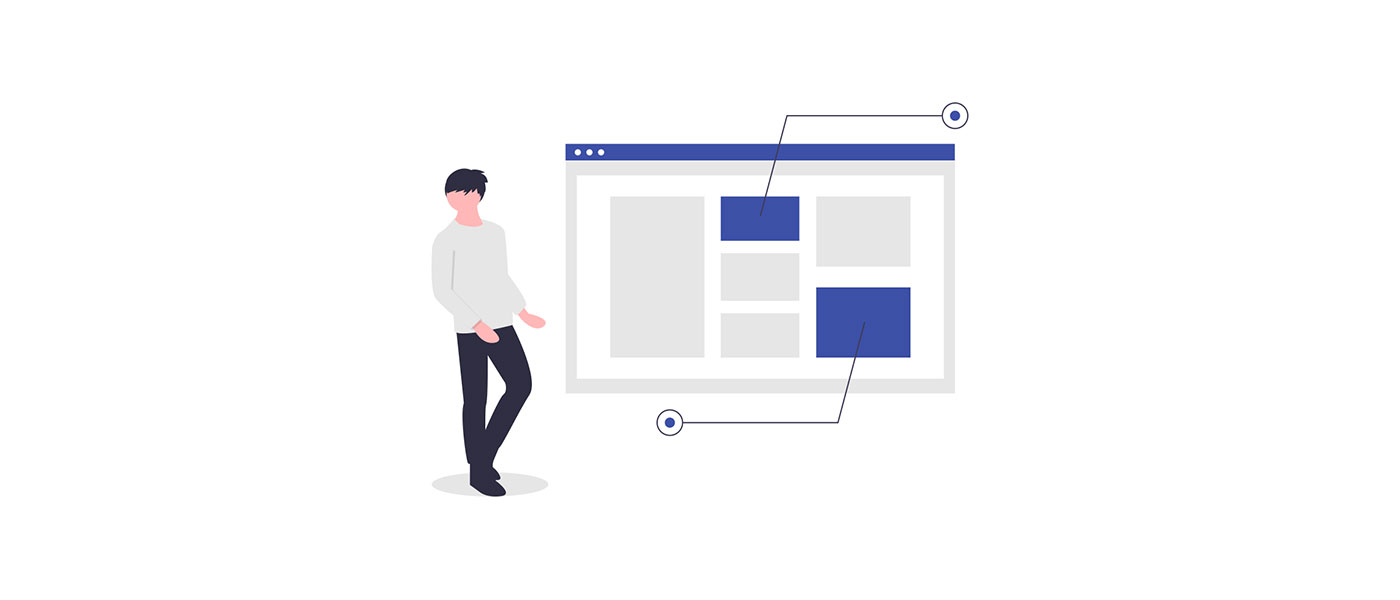
CSS belongs to a website like the sun belongs to summer. There are many things you should consider or in another way improve and optimize. I will tell you my CSS tricks!
Cascading Style Sheets exist on almost every website on the Internet and are one of the basics of the Internet. Years of development give you the potential to optimize your own websites and web applications again and again.
I have summarized some CSS tricks and tricks to make bad-practice and good-practice procedures clear.
Note: These CSS tricks are only my personal opinion. They are not fixed rules, but only tricks from my own experience!
1. Style Resets
Each HTML element has some default styles, which should make the element unique. This also determines the area of application and the purpose of the element. Therefore you should use these default styles and not reset them. The p
tag is a good example to explain the problem.
/* No-Go */ /* Default Style */ p { display: block; margin-top: 1em; margin-bottom: 1em; margin-left: 0; margin-right: 0; } /* Wrong: Our custom Style */ p { display: inline-block; margin: 0; }
We would overwrite the default display
and margin
attribute. A span
element is more appropriate here, since it has no styles by default.
It better be:
/* Correct: Our custom Style */ span { display: inline-block; margin: 0; }
Of course we have to adjust styles for many elements and sometimes overwrite them, otherwise they would not match the CD (corporate design) and every page would look identical.
However, you can already pay attention to it a little bit. This saves CSS code and thus important (milli-) seconds when loading the page.
Here you can find a list with the default styles for all HTML tags.
2. Bad class names
This CSS trick is very important when other developers need to modify the code. Like well-chosen comments, class names are an important step for readable code.
On this point we must distinguish between three errors.
2.1 Too short – meaningless
If class names are chosen too short, they are usually meaningless. What can we do with f
? Right: nothing! Therefore always choose meaningful class names and try to use as many helper classes as possible. You should not do it this way:
/* No-Go */ .f { color: red; } .a { margin: 1rem 3rem 2rem 3rem; } .cf { display: none; }
This is what meaningful helper classes would look like:
.red-color { color: red; } .margin-small-big { margin: 1rem 3rem 2rem 3rem; } .display-none { display: none; }
2.2 Redundant
These class names are understandable but completely superfluous. We can select HTML elements by the element name section
or span
and do not need an additional class with the same name. The following is a no-go:
/* No-Go */ section.section { min-height: 80vh; } span.span { color: red; font-size: 12px; }
It would be better that way:
section { min-height: 80vh; } span { color: red; font-size: 12px; }
2.3 Confusing
Confusing class names are the worst. When I read no-margin
in the code, I also assume that the margin in the CSS is set to zero. Also with color-red
, everyone assumes that the background color or font color is set to red. It should not look like this:
/* No-Go */ p.color-red { color: green; } .no-margin { margin: 3rem 0.5rem; }
Class names could look like this, which does not confuse:
p.color-green { color: green; } .margin-big-small { margin: 3rem 0.5rem; }
3. Important Keyword
We all know it: A style that cannot be changed because it is often overwritten or comes from a plugin. Then we like to use the little magic word !important
in our CSS.
It causes this style to always work and the problem is supposedly solved. But this is the beginning of a vicious circle. The next time you want to change it and are too lazy to search again, you use the keyword again and eventually there is complete chaos.
Therefore you should always think and search carefully before you use the keyword. Sometimes it is indeed unavoidable, but more often it can be solved differently.
So don’t do anything like that:
/* No-Go */ span.foobar { color: red!important; margin-top: 4rem!important; font-size: 12px!important; border-bottom: 1px solid #000!important; }
4. Empty selectors
Of all CSS tricks, this is probably the least important one, but at the same time the one that can be implemented most easily.
We’re talking about empty selectors. So selectors that do not contain any attributes. These selectors always cause a few bytes of memory to be lost.
If this often occurs in the code and we have a lot of large CSS files, it can affect the loading time of your page – even if only minimally.
Therefore such things have no place in CSS:
/* No-Go */ p { } span { } .no-margin { } footer { } footer > a { }
5. Overwriting Styles
We have already talked about the keyword “!important”. With it we can overwrite styles, so that this CSS rule always applies. If we use this too often we will have big problems with later changes. But even without the keyword we should avoid overwriting for the most part.
You should avoid that:
/* No-Go*/ p { font-size: 2em; color: #FFF; } /* ..other code.. */ p { font-size: 2.2em; line-height: 20px; } /* ..other code.. */ p { font-size: 1.8em; }
But you can easily avoid this mistake. Instead of always adding a new style, you can simply search for an occurrence in your CSS files for CTRL + F and change it accordingly.
Thus we save code, thus memory space and the file remains also clearer and smaller.
It could look that way:
p { font-size: 1.8em; }
6. Absolute positioning
I love absolute positioning. It can be really helpful in many cases (e.g. CSS buttons) and is also inevitable and good in some cases.
However, there are (allegedly) still people who position each element absolutely. There are many reasons why this should not be done. First of all, it is much more complex to position each element pixel-exactly at a certain position. A lot of paperwork, a lot of trial and error, and it usually looks messy.
Also, it is much more difficult to make a page responsive and you have to insert a lot of breakpoints to make the page look good at any resolution. Why should you make life harder than it is…? But like I said: there are also many good applications for absolute positioning.
7. Too much CSS
A problem what I see for example with ready-made WordPress themes. You already have a lot of CSS code (including a lot of unnecessary code) and then you even add your own code. So the chaos is complete.
Many of the tricks listed here will be effective. Overwriting, empty selectors, frequent use of “!important” and above all: confusing! Therefore you should directly remove or rewrite all code that is not needed or that you are modifying.
But also for other projects you should write minimal code. It makes a lot of things easier and minimalism has become modern and beautiful.
8. Outline
The CSS outline is often simply removed because it does not look nice at first. Yes, I have done it that way for a long time. The outline always appears when an element gets the property focus.
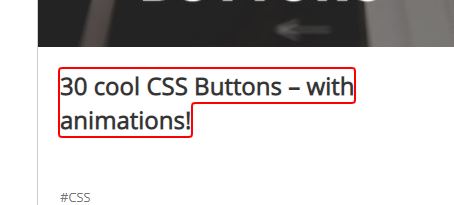
But I had to realize that the outline is very important. Important for accessibility on the Internet.
Ever tried to navigate a website with only the tab key? Not, then it is high time. Just find any website (maybe your own) and try it out.
If the outline was removed, you will see that it is impossible.
To make the outline look chic here are some CSS outline ideas. And here you get more information why you should not remove the outline.
What do we learn from this?
So there are many points you can consider to write clear and performant CSS. You never stop learning and can always improve your “code”.
I also know that you can’t always follow these CSS tricks, but every change is a step in the right direction. 🙂
You can now directly try to apply my CSS tricks by creating your own WYSIWYG editor with HTML, CSS and JavaScript.
What did you think of this post?


-
Pingback: Programming Reading Position Indicator (CSS & JavaScript)
-
Pingback: Programming Reading Position Indicator with jQuery » webdeasy.de
-
Pingback: The most important HTML Meta Tags: An overview » webdeasy.de