JavaScript Arrays – JS Array Functions Explained (Cheatsheet)
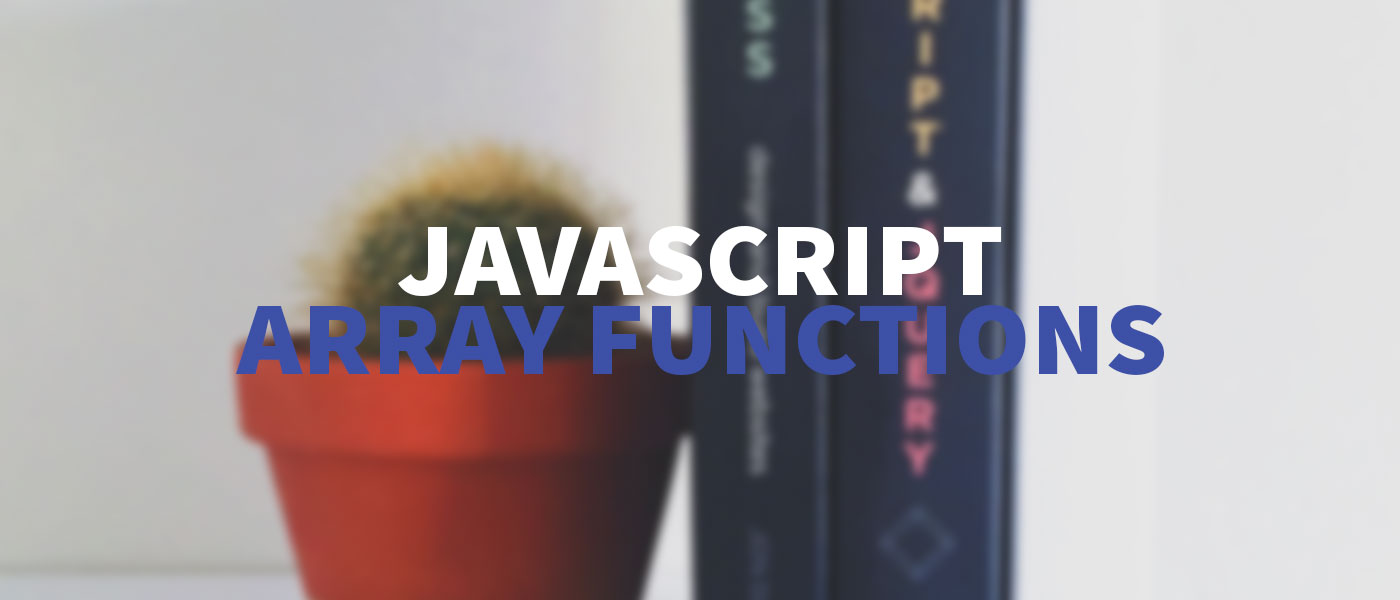
JavaScript arrays are list-like structures, can store data and are necessary for almost every program. I explain the most important JavaScript array functions here.
Besides all the standard array functions, like push()
and pop()
, there are also many useful functions that can save you a lot of time. Especially with the introduction of ES6 and the Arrow Functions even more useful functions have been added.
- Basics: What are JavaScript Arrays?
- 1. Array.map() - Map functions to elements
- 2. Array.filter() - Filter elements in the array
- 3. Array.forEach() - Loop over all array elements
- 4. Array.indexOf() - Find index of an element
- 5. Array.find() - Search elements in the array
- 6. Array.sort() - Sort values
- 7. Array.reverse() - Reverse everything
- 8. Array.concat() - Interrelate arrays
- Conclusion
Basics: What are JavaScript Arrays?
JavaScript arrays are to be understood like arrays in most other known programming languages. They allow one to store multiple values in a single variable, query, delete or modify them there.
Arrays are indispensable in hardly any program, and they are also an important part of JavaScript programming.
In addition to standard functions such as adding (.add()
) or deleting (.splice()
) individual elements in an array, JavaScript provides many useful functions for performing operations on and with arrays.
If you want to know more about the basics, you can check out this article. But we’ll go straight to the most useful features.
1. Array.map() – Map functions to elements
In this function, a desired operation is performed on each element in the array – this is also called mapping. Thereby we get back a new array with the changed entries. This saves us from programming a loop and in the best case we can implement our function as a one-liner.
For clarity, we have an element with fruits that we want to change.
let fruits = [ 'banana', 'apple', 'avocado', 'cherry', 'grapefruit', 'melon' ]; const fruitsLongerSix = fruits.map(fruit => 'Fruit: ' + fruit); console.log(fruitsLongerSix); // Output: ["Fruit: banana", "Fruit: apple", "Fruit: avocado", "Fruit: cherry", "Fruit: grapefruit", "Fruit: melon"]
If our statement gets more complicated, we can also outsource the callback in a separate function. This would look like this:
let fruits = [ 'banana', 'apple', 'avocado', 'cherry', 'grapefruit', 'melon' ]; const fruitsLongerSix = fruits.map(addPrefix); function addPrefix(entry) { return 'Fruit: ' + entry; } console.log(fruitsLongerSix); // Output: ["Fruit: banana", "Fruit: apple", "Fruit: avocado", "Fruit: cherry", "Fruit: grapefruit", "Fruit: melon"]
2. Array.filter() – Filter elements in the array
This function creates a new array with all elements that pass the implemented test. This means we can filter our array, just as we want. This is a simple and clean way to filter list items.
We have here again our array with fruits and need only entries with an “o” in the name. By a single line we can implement this function.
let fruits = [ 'banana', 'apple', 'avocado', 'cherry', 'grapefruit', 'melon' ]; let filtered = fruits.filter(fruit => fruit.indexOf('o') > -1); console.log(filtered); // Output: ["avocado", "melon"]
If the filtering becomes a bit more complex, we can again outsource the callback of the filter() function to an extra function as well, as the following example shows.
let fruits = [ 'banana', 'apple', 'avocado', 'cherry', 'grapefruit', 'melon' ]; let filtered = fruits.filter(getO); function getO(entry) { return entry.indexOf('o') > -1; } console.log(filtered); // Output: ["avocado", "melon"]
3. Array.forEach() – Loop over all array elements
This JavaScript array function can replace our for
loop. By less code we can iterate over each element of an array. In principle, it works like the map()
function, except for the difference that no new array is created here.
This is what a normal loop looks like. Much simpler and clearer than a for
loop, isn’t it?
let fruits = [ 'banana', 'apple', 'avocado', 'cherry', 'grapefruit', 'melon' ]; fruits.forEach((fruit) => { console.log(fruit); }); // Output: // banana // apple // avocado // cherry // grapefruit // melon
Sometimes we need an additional index, or as I like to call it “counter”. This can also be realized with this function. For this we specify an additional variable index in the function header.
let fruits = [ 'banana', 'apple', 'avocado', 'cherry', 'grapefruit', 'melon' ]; fruits.forEach((fruit, index) => { console.log(index, fruit); }); // Output: // 0 "banana" // 1 "apple" // 2 "avocado" // 3 "cherry" // 4 "grapefruit" // 5 "melon"
4. Array.indexOf() – Find index of an element
The function returns the index – the position – of an element in an array. So we can also use it to check whether a certain element is in the array.
If we output the result of the function, we get the respective index back. If an element is not present, we get -1
back.
let fruits = [ 'banana', 'apple', 'avocado', 'cherry', 'grapefruit', 'melon' ]; console.log(fruits.indexOf('banana')); // Output: 0 (it's the first index) console.log(fruits.indexOf('cherry')); // Output: 3 onsole.log(fruits.indexOf('toast')); // Output: -1
Furthermore, we can use this query to check if there is a certain element in the array:
if (fruits.indexOf('avocado') > -1) { console.log('We have an avocado!'); }
5. Array.find() – Search elements in the array
With this function we can also check if there is a certain element in the array. It always returns us the first occurrence in the array that matches our condition.
Our statement is: “Return me an element with “o” in its name”. Thereby we get the first result in our result
variable.
let fruits = [ 'banana', 'apple', 'avocado', 'cherry', 'grapefruit', 'melon' ]; const result = fruits.find((fruit) => { return fruit.indexOf('o') > -1; }); console.log(result); // Output: avocado
Again, we can offload more complex instructions to an extra callback function.
6. Array.sort() – Sort values
This function can sort our JavaScript array. So we don’t need to program our own sorting algorithm.
This function converts the elements of the array into strings and compares them by their UTF-16 code points. Therefore strings starting with numbers will always be placed before all string elements.
let fruits = [ 'banana', 'apple', 'avocado', 'cherry', 'grapefruit', 'melon' ]; let sortedFruits = fruits.sort(); console.log(sortedFruits); // Output: ["apple", "avocado", "banana", "cherry", "grapefruit", "melon"]
In addition, a callback can also be specified here, where you specify your own compare function by which the elements are to be sorted.
For example, you can easily sort the elements in descending order (Descending) instead of ascending order (Ascending). The return value of the callback must always be 1
or -1
.
let fruits = [ 'banana', 'apple', 'avocado', 'cherry', 'grapefruit', 'melon' ]; let sortedFruitsDESC = fruits.sort(sortDESC); function sortDESC(a, b) { return a < b ? 1 : -1; } console.log(sortedFruitsDESC); // Output: ["melon", "grapefruit", "cherry", "banana", "avocado", "apple"]
Of course, the same works for other data types, like numbers or objects. This can then look like this.
let fruits = [ {name: 'banana', amount: '2'}, {name: 'apple', amount: '22'}, {name: 'avocado', amount: '404'}, {name: 'cherry', amount: '83'}, {name: 'grapefruit', amount: '26'}, {name: 'melon', amount: '42'} ]; let sortedFruitsByAmount = fruits.sort(sortDESC); function sortDESC(a, b) { return a.amount > b.amount ? 1 : -1; } console.log(sortedFruitsByAmount); // Output: // 0: {name: "banana", amount: "2"} // 1: {name: "apple", amount: "22"} // 2: {name: "grapefruit", amount: "26"} // 3: {name: "avocado", amount: "404"} // 4: {name: "melon", amount: "42"} // 5: {name: "cherry", amount: "83"}
7. Array.reverse() – Reverse everything
This function is explained quite quickly: the order of the elements is simply reversed.
let fruits = [ 'banana', 'apple', 'avocado', 'cherry', 'grapefruit', 'melon' ]; let reversedFruits = fruits.reverse(); console.log(reversedFruits); // Output: ["melon", "grapefruit", "cherry", "avocado", "apple", "banana"]
There are no further parameters, but you will need this function sooner or later. This saves you 7 lines of code.
function reverse(array) { let reverse = []; for(let i = array.length - 1; i >= 0; i--) { reverse.push(array[i]); } return reverse; }
8. Array.concat() – Interrelate arrays
With this method you can concatenate two or more arrays. This can be useful, for example, if you evaluate filter functions and then output all values bundled in an array to the visitor.
let fruitsOne = [ 'banana', 'apple', 'avocado' ]; let fruitsTwo = [ 'cherry', 'grapefruit', 'melon' ]; let concatedFruits = fruitsOne.concat(fruitsTwo); console.log(concatedFruits); // Output: ["banana", "apple", "avocado", "cherry", "grapefruit", "melon"]
Conclusion
As you can see there are very useful JavaScript array functions. Many of them can be implemented with just a few lines of code and deliver super great results.
Moving on, here are the best examples to learn Vue.js, right? 😉
What did you think of this post?

