Programming JavaScript Equalizer
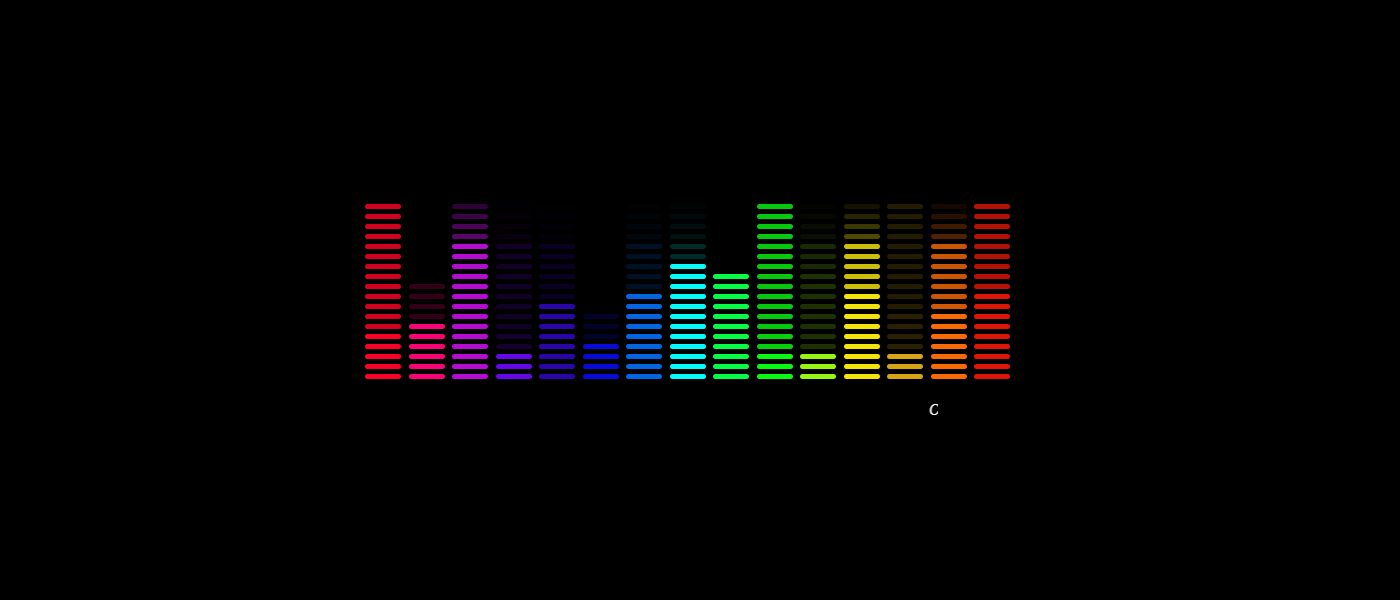
Simply as a design element or functional, this equalizer is a real eye-catcher! Here I explain to you how you can program this equalizer yourself with pure JavaScript.
In the music industry, equalizers are responsible for displaying and visualizing the highs and lows of a song. And that’s exactly what we’re demonstrating here, just… with random values.
1. The HTML
In the first step we create the single bars .equalizer-bar
and the lowest bar per bar as span
.
<div class="equalizer"> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> <div class="equalizer-bar"> <span></span> </div> </div>
Then we insert the scrolling text with the help of the marquee
tag.
<div class="song-info"> <marquee>Currently playing: Awesome song - WebDEasy ft. codepen.io - Duration: Endless - File: 404 not found</marquee> </div>
2. The Styling
I did the complete styling with SASS (Syntactically Awesome Stylesheets). If you just want to do the styling, you can use this tool to convert it to normal CSS.
I set the font, background and basic settings for these three elements.
body { font-family: arial, sans-serif; background-color: #000; width: 100%; height: calc(100vh - 4rem); margin: 0; } .equalizer { display: flex; justify-content: center; align-items: center; height: 10rem; margin-top: 4rem; } .song-info { color: #FFF; width: 25rem; font-style: italic; font-size: 12px; margin: .5rem auto; }
Then it’s on to the main element: the equalizer and its bars and beams.
Here it is important that the single span
elements get a transition of 200 milliseconds, because the effect should come into its own later. I tried different values and got a good result.
The individual bars all get different colors, as with many real equalizers.
.equalizer-bar { width: 1.8rem; margin: 0 3px; display: flex; flex-direction: column-reverse; span { display: block; height: 4px; border-radius: 2px; width: 100%; margin: 2px 0; background-color: #FFF; transition: .2s ease all; opacity: 1; } &:nth-child(1) span { background: #fc0127; } &:nth-child(2) span { background: #fb0275; } &:nth-child(3) span { background: #b50cd3; } &:nth-child(4) span { background: #6407e9; } &:nth-child(5) span { background: #2a06a9; } &:nth-child(6) span { background: #080ad7; } &:nth-child(7) span { background: #0265e2; } &:nth-child(8) span { background: #03fcfc; } &:nth-child(9) span { background: #02fe46; } &:nth-child(10) span { background: #05fb0f; } &:nth-child(11) span { background: #97f611; } &:nth-child(12) span { background: #f5e506; } &:nth-child(13) span { background: #d7a414; } &:nth-child(14) span { background: #fc6b02; } &:nth-child(15) span { background: #df1506; } }
3. Enlivening the Equalizer with JavaScript
Let’s now come to the most exciting part in my eyes: Programming.
In the first step we define a constant with the bar height. This is the maximum number of bars, i.e. the actual height of the equalizer.
Then we call the function addBarSpans()
. This function inserts the number of spans into each bar. This has saved us a lot of typing in HTML and is only used for preparation.
const MAX_BAR_HEIGHT = 18; addBarSpans(); // Add the default spans function addBarSpans() { const bars = document.getElementsByClassName('equalizer-bar'); let html = ''; for(let j = 0; j < MAX_BAR_HEIGHT; j++) { html += '<span></span>'; } for(let i = 0; i < bars.length; i++) { bars[i].innerHTML = html; } }
Next we’ll write two help functions which we’ll need later.
The getActiveSpans(spans)
function returns the number of spans that are visible – i.e. active. We also have the function getRandomHeight()
, which returns a random number between one and the maximum height of our bars.
// Returns the number of active spans function getActiveSpans(spans) { let counter = 0; for(let i = 0; i < spans.length; i++) { if(spans[i].style.opacity > 0) counter++; } return counter; } // Returns a random number between 1 and 20 function getRandomHeight(maxBarHeight) { return Math.round(Math.random() * (maxBarHeight - 1)) + 1; }
Now comes our main program. We call the function setRandomBars()
every 200 milliseconds. This “loop” then runs endlessly.
The function has a loop over all bars (first marked line) and a loop over all bars in this bar (second marked line).
All necessary values are determined beforehand (lines 11-13). All span
elements that are higher than the new height are hidden, all others are displayed.
Afterwards (lines 23-27) the five upper bars are calculated and the visibility (opacity
) is set descending. This results in a more beautiful and smoother upward effect.
setInterval(() => { setRandomBars(); }, 200); // Main programm (repeats) function setRandomBars(maxBarHeight) { const bars = document.getElementsByClassName('equalizer-bar'); for(let i = 0; i < bars.length; i++) { let spans = bars[i].getElementsByTagName('span'); let activeSpanCount = getActiveSpans(spans); let newHeight = getRandomHeight(MAX_BAR_HEIGHT); for(let j = 0; j < spans.length; j++) { if(newHeight > activeSpanCount) { spans[j].style.opacity = '1'; } else if(j > newHeight) { spans[j].style.opacity = '0'; } // set little opacity let upperSpan = MAX_BAR_HEIGHT - j; if(newHeight > MAX_BAR_HEIGHT-5 && upperSpan < 5) { spans[j].style.opacity = '0.' + upperSpan; } } } }
4. Conclusion
This equalizer is versatile and maybe you have an API that you can connect to this equalizer to make it work – and not just random values.
Thank you for reading this page and I’m looking forward to your feedback! 🙂
What did you think of this post?


Hallo Lorenz.I’m a gamer and music streamer and I REALLY love your Equalizer program, and with your permission I would like used it on my stream. But that’s the problem. You see I’m gamer not programmer, so here some of my question.Can I get your permission to used your equalizer on my stream?What software should I use to edit this equalizer? I only have Notepad++ How to make your equalizer transparent on my stream?How to edit to make six bar only and export as a .gif file to be my emote?How to connect your equalizer with my music playlist or connect with my voice?I really appreciate for your reply and please send your reply to my email. Thank you so much for your time.🙏
Sounds cool!
This is a VISUALIZER, not an EQUALIZER
It should look like an Equalizer, and it does.. 😉