RegEx Validation: Cheatsheet of the most common regular expressions for validation (+ HowTo)
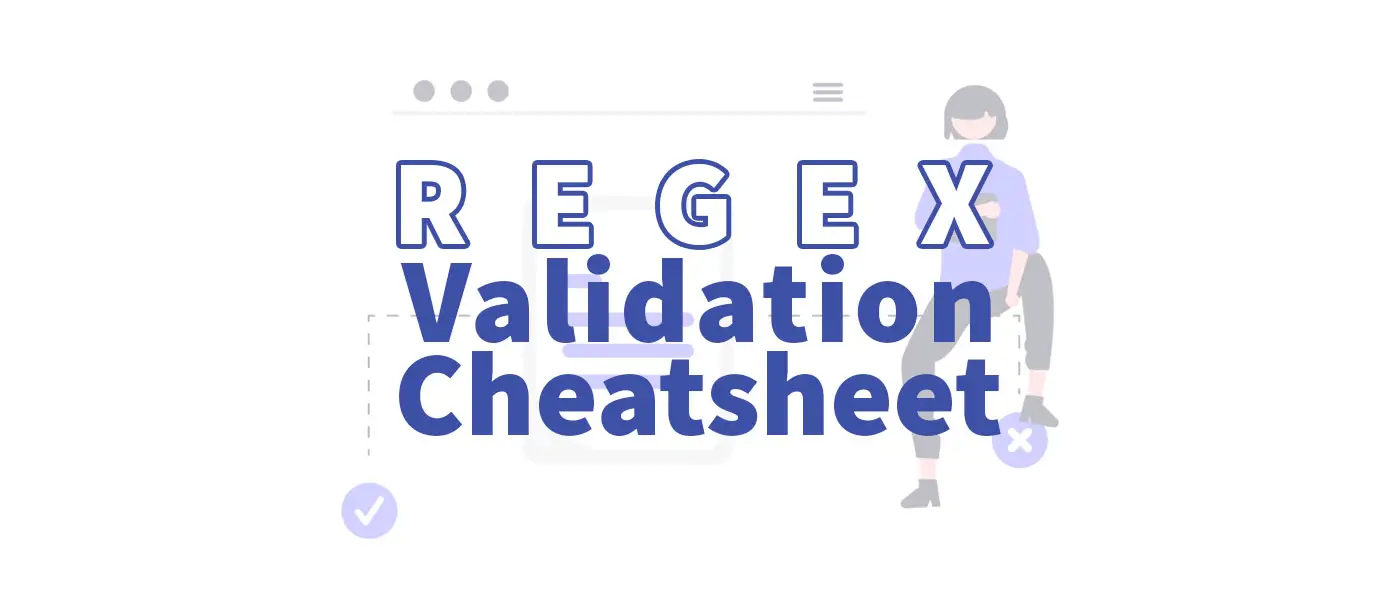
Regular expressions are often used to validate user input. These are repeated when validating the same input. Therefore I have created a list with the most important RegEx strings.
Just save this article and copy the corresponding regular expression into your next project where you need it.
For all RegEx validations there are infinite possibilities in the implementation. I have tested all the ones shown here extensively.
To test regular expressions more easily and not run into trial and error there are several tools online. I have linked my favorite RegEx Validator here:
Validate date & time
Date in the format YYYMMDD (0-9999 years)
(?<!\d)(?:(?:(?:1[6-9]|[2-9]\d)?\d{2})(?:(?:(?:0[13578]|1[02])31)|(?:(?:0[1,3-9]|1[0-2])(?:29|30)))|(?:(?:(?:(?:1[6-9]|[2-9]\d)?(?:0[48]|[2468][048]|[13579][26])|(?:(?:16|[2468][048]|[3579][26])00)))0229)|(?:(?:1[6-9]|[2-9]\d)?\d{2})(?:(?:0?[1-9])|(?:1[0-2]))(?:0?[1-9]|1\d|2[0-8]))(?!\d)
Date in the format YYYY-MM-DD
^\d{4}\-(0?[1-9]|1[012])\-(0?[1-9]|[12][0-9]|3[01])$
Date in the format DD.MM.YYYY (without leading zeros)
^[0-3]?[0-9][\/.][0-3]?[0-9][\/.](?:[0-9]{2})?[0-9]{2}$
Date in the format DD.MM.YYYY (with leading zeros)
^[0-3][0-9][\/.][0-3][0-9][\/.](?:[0-9][0-9])?[0-9][0-9]$
Date in M/D/YYY format
^(0?[1-9]|[12][0-9]|3[01])[\/\-](0?[1-9]|1[012])[\/\-]\d{4}$
Time in HH:MM format (24 hours format)
^([0-1]?[0-9]|2[0-3]):[0-5][0-9]$
Time in HH:MM:SS format (24 hours format)
^([0-1]?[0-9]|2[0-3]):[0-5][0-9]:[0-5][0-9]$
Time in HH:MM format (12 hours format)
^(0?[1-9]|1[0-2]):[0-5][0-9]$
Time in HH:MM:SS format (12 hours format)
^(0?[1-9]|1[0-2]):[0-5][0-9]:[0-5][0-9]$
Time in H:MM AM/PM format
^((1[0-2]|0?[1-9]):([0-5][0-9]) ?([AaPp][Mm]))$
Time in the format H:MM:SS AM/PM
^((1[0-2]|0?[1-9]):([0-5][0-9]):([0-5][0-9]) ?([AaPp][Mm]))$
Validate password
Only numbers and letters (at least 8 characters)
^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)[a-zA-Z\d]{8,}$
Numbers, upper and lower case letters (at least 12 characters)
^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&#\^]{12,}$
Validate phone numbers & mobile numbers
Recognizes most popular formats (different countries)
(\(?([\d \-\)\–\+\/\(]+)\)?([ .\-–\/]?)([\d]+))
Validate email address
Only email addresses from specific domains (here *@gmail.com and @hotmail.com)
^([\w\-\.]+@(?!gmail.com)(?!hotmail.com)([\w\- ]+\.)+[\w-]{2,4})?$
For all domains
^[a-zA-Z0-9.!#$%&'*+\/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$
Validate URL
Only URLs with FTP protocol
^(ftp:\/\/)[a-z0-9]+([\-\.]{1}[a-z0-9]+)*\.[a-z]{2,5}(:[0-9]{1,5})?(\/.*)?$
Standard URLs (only in the following format: http(s)://example.com/ , including ports)
^(http:\/\/|https:\/\/)[a-z0-9]+([\-\.]{1}[a-z0-9]+)*\.[a-z]{2,5}(:[0-9]{1,5})?(\/.*)?$
All possible URL formats (including IP addresses and anchors)
^(?:http(s)?:\/\/)?[\w.-]+(?:\.[\w\.-]+)+[\w\-\._~:/?#[\]@!\$&'\(\)\*\+,;=.]+$
Implementation in different programming languages
Validation in different programming languages always looks slightly different. However, in general it is identical.
In all examples you just replace the variable regex
with one of the above and the stringToValidate
should contain a string with the respective user input.
RegEx Validierung in JavaScript
let stringToValidate = "ZH*EnD?vv9Xf&y8"; // e.g. value from a textarea let regex = /^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&#\^]{12,}$/; // attention: no quotes! if (stringToValidate.match(regex)) { // valid input } else { // invalid input }
RegEx Validierung in PHP
$stringToValidate = "ZH*EnD?vv9Xf&y8"; $regex = "/^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&#\^]{12,}$/"; // attention: add a slash at the begin and the end if (preg_match($regex, $stringToValidate)) { // valid input } else { // invalid input }
RegEx Validierung in Java
String stringToValidate = "ZH*EnD?vv9Xf$y8"; String regex = "^(?=.*[a-z])(?=.*[A-Z])(?=.*\\d)(?=.*[@$!%*?&])[A-Za-z\\d@$!%*?&#\\^]{12,}$"; if (stringToValidate.matches(regex)) { // valid input } else { // invalid input }
What validation is still missing here? Feel free to write me a comment! 🙂
What did you think of this post?


-
Pingback: TOP 20 VSCode Extensions to increase your productivity!