WordPress: Pass PHP Variables to JavaScript
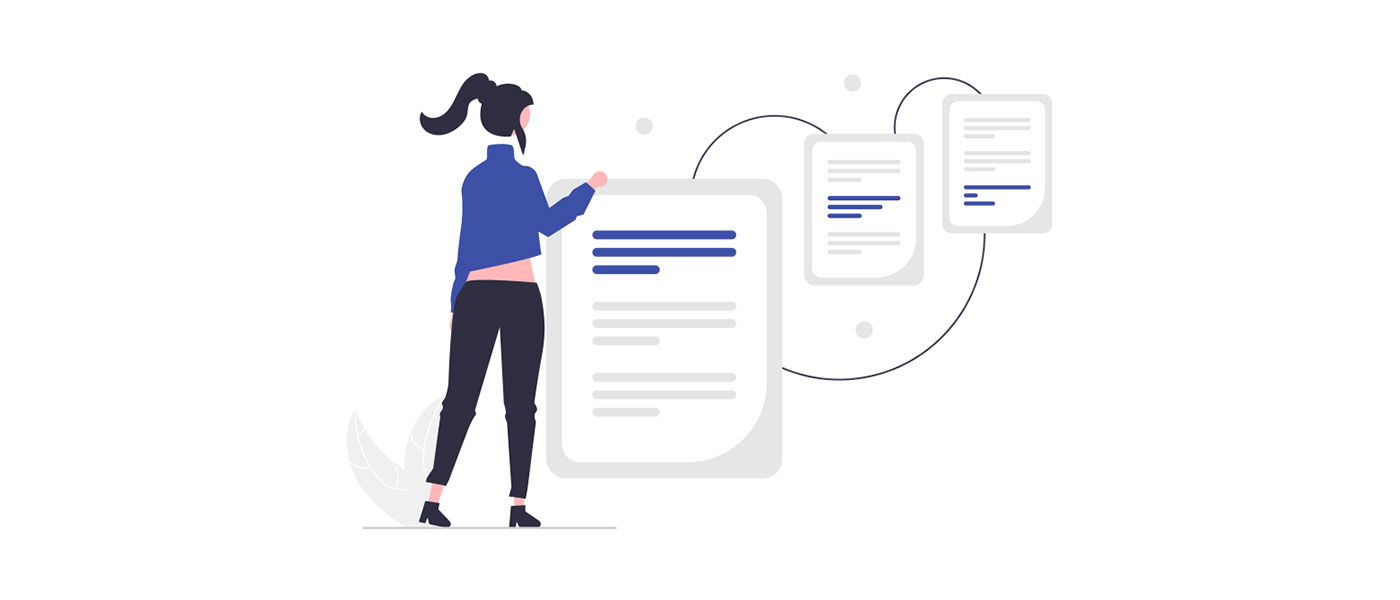
WordPress provides a simple, secure and fast way to pass arbitrary PHP variables to a JavaScript file.
Often in WordPress PHP variables are also needed in a JavaScript file. Of course you could now simply make a <script>
tag in the HTML and create the variables there.
However, WordPress comes with a simple function wp_localize_script()
that does the job for you. And that’s how simple it works.
1. Embed JavaScript file in WordPress
In WordPress, JavaScript files should always be included using wp_enqueue_script()
instead of an HTML <script>
tag. To do this, you can use the following code snippet and add it to your functions.php.
// functions.php function register_js_files() { wp_enqueue_script("my_own_script", get_template_directory_uri() . "/js/script.js"); } add_action("wp_enqueue_scripts", "register_js_files");
The first parameter is an arbitrary name, which may not be assigned twice. The second parameter is the path to your JavaScript file. In this case it is located in the theme folder under js/script.js. (Path to the child theme: get_stylesheet_directory_uri()
).
Optionally, you can specify additional parameters: The scripts to be loaded beforehand (e.g. jQuery), a version string to be appended as a parameter to the JavaScript file and a boolean whether the script should be loaded in the head or in the footer of the page. With these parameters, the line could look like this:
wp_enqueue_script("my_own_script", get_template_directory_uri() . "/js/script.js", array("jquery"), "1.3.3", true);
Here you can find a lot of useful snippets for WordPress 🙂
2. Pass WordPress PHP variables to JavaScript
After the wp_enqueue_script()
function we add the marked lines where the PHP data is passed.
// functions.php function register_js_files() { wp_enqueue_script("my_own_script", get_template_directory_uri() . "/js/script.js"); wp_localize_script("my_own_script", "WPVars", array( "your_data" => "HIHI FUNNY DATA", "blog_name" => get_bloginfo("name"), "time" => time(), "images_folder" => get_template_directory_uri() . "/images", ) ); } add_action("wp_enqueue_scripts", "register_js_files");
The wp_localize_script()
function expects 3 parameters:
- $handle: The name of the script which should get the variables (
my_own_script
in our case) - $object_name: The variable name that the JavaScript object with our data should have
- $l10n: The actual data as PHP array (multidimensional)
3. Using variables in JavaScript
In the included JavaScript file we can now access the PHP variables with our chosen object name.
console.log(WPVars.your_data); console.log(WPVars.blog_name); console.log(WPVars.time); console.log(WPVars.images_folder);
What you do now with these variables is up to you – have fun with it! 🙂
If you want to know how to transfer data between WordPress and browser dynamically (e.g. for pagination, live search and dynamic content reloading), check out my post on Ajax in WordPress.
What did you think of this post?


-
Pingback: How To Create & Access Environment Variables In WordPress Project (with Divi Plugin) Resident On AWS LightSail - Programming Questions And Solutions Blog
-
Pingback: Cómo crear y acceder a variables de entorno en un proyecto de WordPress (con Divi plugin) que residen en AWS LightSail - javascript en Español
-
Pingback: How to use Ajax correctly in WordPress